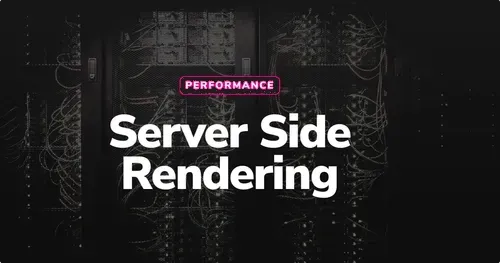
SSR with Astro - Part 1, Introduction
Introduction for SSR with Astro, showing Astro's special features over other default solutions for SSR
Wed, May 01, 2024
-->
We’ll discuss how we’ll deal with our adapter’s output strategy and how to control your pages and components rendering, but take note that I’ll refer a lot to Astro’s docs, because it’s the original source of information.
We’ve got 2 options:
---
// src/pages/*.astro
export const prerender = false;
---
---
// src/pages/*.astro
export const prerender = true;
---
So let’s talk more about specifics…
In most of our applications, we have different needs for our pages, the decision is yours. A lot of times, your page is static by default, like your about and authentication pages.
But there are some cases where you need your page to be server-rendered:
---
// src/pages/partner/history
// Canceling prerender, because we use hybrid output so that we can read the cookie and act.
export const prerender = false;
const isAuthenticated = Astro.cookies.has("accessToken");
if (!isAuthenticated) return Astro.redirect("/partners/auth");
---
---
// GET /api/poet/:id
// src/pages/poet/[id].astro
// Canceling prerender, because we use hybrid output
export const prerender = false;
const { id } = Astro.params;
if (!id) Astro.redirect("/");
else await fetchPoet(id);
---
Until now, I didn’t meet any cases that made it a must to server-render a page, I prefer to make static rendering my default, it’s just enough.
Now, let’s talk about how we can use Astro’s client-directives to control which component is hydrated in the client or not and when it’ll be hydrated, but it can only apply to other UI frameworks like Vuejs and React. Every directive has a priority level, so Astro knows which is more needed.
Astro has five directives:
The same component can have different client-directive on different pages>
As an example from my project “Adeeb”, I have a component where I show most of my HTTP errors messages for users that take a client-directive on pages like the authentication page, order page and history page, so that it reacts to a failed login or invalid order:
<!-- ........... -->
<HttpPopUp client:idle slot="http-popup" />
<!-- ........... -->
But it doesn’t take any directives in /poem/[id] or /poet/[id] pages, so it reacts to non-existing IDs:
<!-- ........... -->
<HttpPopUp slot="http-popup" />
<!-- ........... -->
Another component like SelectPrints, where I persist data on SessionStorage, uses a client-only directive:
<!-- src/pages/index.astro -->
<SelectedPrints client:only="vue" />
Astro gives you full control over your components, it only depends on your architecture and whether you make the right decision.